🍭MUI Global styleOverrides
TSS Support MUI Global style overrides from createTheme
out of the box. Previously in material-ui v4 it was: global theme overrides.
By default, however, only the theme
object is passed to the callbacks, if you want to pass the correct props
, and a specific ownerState
have a look at the following example:
import { tss } from "tss-react/mui";
export type Props = {
className?: string;
classes?: Partial<ReturnType<typeof useStyles>["classes"]>;
lightBulbBorderColor: string;
}
function MyComponent(props: Props) {
const { className } = props;
const [isOn, toggleIsOn] = useReducer(isOn => !isOn, false);
const { classes, cx } = useStyles({
muiStyleOverridesParams: {
props,
"ownerState": { isOn }
}
});
return (
<div className={cx(classes.root, className)} >
<div className={classes.lightBulb}></div>
<button onClick={toggleIsOn}>{`Turn ${isOn?"off":"on"}`}</button>
<p>Div should have a border, background should be white</p>
<p>Light bulb should have black border, it should be yellow when turned on.</p>
</div>
);
}
const useStyles = tss
.withName("MyComponent")
.create({
root: {
border: "1px solid black",
width: 500,
height: 200,
position: "relative",
color: "black"
},
lightBulb: {
position: "absolute",
width: 50,
height: 50,
top: 120,
left: 500/2 - 50,
borderRadius: "50%"
}
});
Declaration of the theme:
import { createTheme } from "@mui/material/styles";
import { ThemeProvider } from "@mui/material/styles";
const theme = createTheme({
components: {
//@ts-ignore: It's up to you to define the types for your library
MyComponent: {
styleOverrides: {
lightBulb: ({ theme, ownerState: { isOn }, lightBulbBorderColor })=>({
border: `1px solid ${lightBulbBorderColor}`,
backgroundColor: isOn ? theme.palette.info.main : "grey"
})
}
}
}
});
render(
<MuiThemeProvider theme={theme}>
{/*...*/}
</MuiThemeProvider>
);
Usage of the component:
import { useStyles } from "tss-react/mui";
function App(){
const { css } = useStyles();
return (
<MyComponent
className={css({ "backgroundColor": "white" })}
classes={{
root: css({
backgroundColor: "red",
border: "1px solid black"
})
}}
lightBulbBorderColor="black"
/>
);
}
Result:
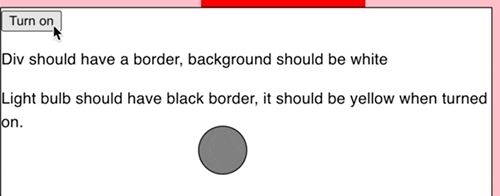
You can see the code here and it's live here (near the bottom of the page).
Last updated
Was this helpful?